Poker Api Javascript
- Poker Api Javascript App
- Poker Api Javascript Server
- Poker Api Javascript Games
- Poker Api Javascript Games
- Poker Api Javascript Download
- Poker Api Javascript App
Shape¶
poker.hand.
Shape
[source]¶An enumeration.
See: Shape
Poker is one of the most popular table games. This free card game will give you an idea of the game, but you won't lose real money so that you can sleep at night! The game of poker originated during the early 19th century. The unique features of poker involve betting depending on the ranking of the cards. Nov 26, 2020 In this post, I will be going over how to build a deck of cards in JavaScript, that can be used for future projects or future games, such as this JavaScript Blackjack game. This is a super quick implementation, and can be done in less than 100 lines of code. Poker.JS is a JS lib extend HTML5 canvas to draw poker card. User should easy to create single poker card via img or canvas, or draw card in big canvas. Deck of Cards API. GitHub Gist: instantly share code, notes, and snippets. We offer over 800 free APIs for developers to develop the next big thing, add yours if you own an API.
Warning
This might be removed in future version for simplify API.
Hand¶
poker.hand.
Hand
(hand)[source]¶General hand without a precise suit. Only knows about two ranks and shape.
Parameters: | hand (str) – e.g. ‘AKo’, ‘22’ |
---|---|
Variables: |
|
rank_difference
¶The difference between the first and second rank of the Hand.
Type: | int |
---|
first
¶Type: | poker.card.Rank |
---|
second
¶Type: | poker.card.Rank |
---|
shape
¶Type: | Shape |
---|
is_broadway
¶
is_connector
¶
is_offsuit
¶
is_one_gapper
¶
is_pair
¶
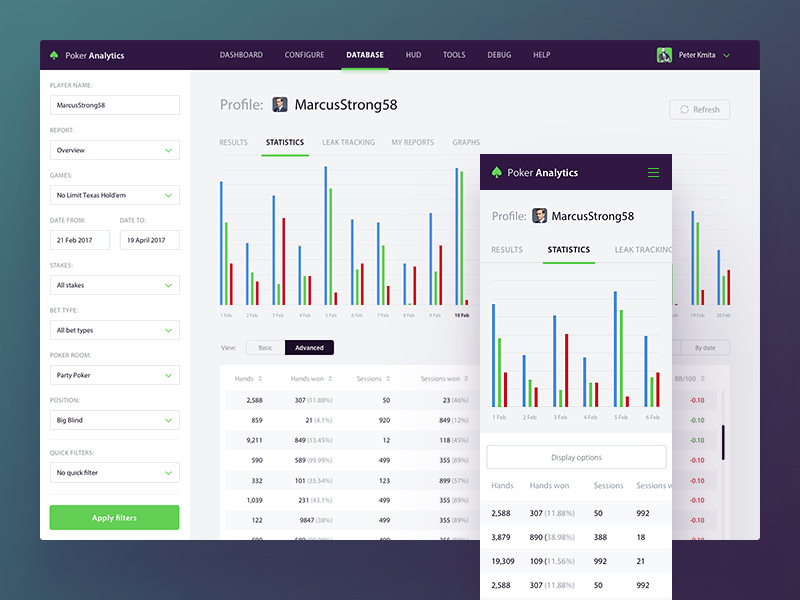
is_suited
¶
is_suited_connector
¶
is_two_gapper
¶
to_combos
()[source]¶
poker.hand.
PAIR_HANDS
= (Hand('22'), Hand('33'), Hand('44'), Hand('55'), Hand('66'), Hand('77'), Hand('88'), Hand('99'), Hand('TT'), Hand('JJ'), Hand('QQ'), Hand('KK'), Hand('AA'))¶Tuple of all pair hands in ascending order.

poker.hand.
OFFSUIT_HANDS
= (Hand('32o'), Hand('42o'), Hand('43o'), Hand('52o'), Hand('53o'), Hand('54o'), Hand('62o'), Hand('63o'), Hand('64o'), Hand('65o'), Hand('72o'), Hand('73o'), Hand('74o'), Hand('75o'), Hand('76o'), Hand('82o'), Hand('83o'), Hand('84o'), Hand('85o'), Hand('86o'), Hand('87o'), Hand('92o'), Hand('93o'), Hand('94o'), Hand('95o'), Hand('96o'), Hand('97o'), Hand('98o'), Hand('T2o'), Hand('T3o'), Hand('T4o'), Hand('T5o'), Hand('T6o'), Hand('T7o'), Hand('T8o'), Hand('T9o'), Hand('J2o'), Hand('J3o'), Hand('J4o'), Hand('J5o'), Hand('J6o'), Hand('J7o'), Hand('J8o'), Hand('J9o'), Hand('JTo'), Hand('Q2o'), Hand('Q3o'), Hand('Q4o'), Hand('Q5o'), Hand('Q6o'), Hand('Q7o'), Hand('Q8o'), Hand('Q9o'), Hand('QTo'), Hand('QJo'), Hand('K2o'), Hand('K3o'), Hand('K4o'), Hand('K5o'), Hand('K6o'), Hand('K7o'), Hand('K8o'), Hand('K9o'), Hand('KTo'), Hand('KJo'), Hand('KQo'), Hand('A2o'), Hand('A3o'), Hand('A4o'), Hand('A5o'), Hand('A6o'), Hand('A7o'), Hand('A8o'), Hand('A9o'), Hand('ATo'), Hand('AJo'), Hand('AQo'), Hand('AKo'))¶Tuple of offsuit hands in ascending order.
poker.hand.
SUITED_HANDS
= (Hand('32s'), Hand('42s'), Hand('43s'), Hand('52s'), Hand('53s'), Hand('54s'), Hand('62s'), Hand('63s'), Hand('64s'), Hand('65s'), Hand('72s'), Hand('73s'), Hand('74s'), Hand('75s'), Hand('76s'), Hand('82s'), Hand('83s'), Hand('84s'), Hand('85s'), Hand('86s'), Hand('87s'), Hand('92s'), Hand('93s'), Hand('94s'), Hand('95s'), Hand('96s'), Hand('97s'), Hand('98s'), Hand('T2s'), Hand('T3s'), Hand('T4s'), Hand('T5s'), Hand('T6s'), Hand('T7s'), Hand('T8s'), Hand('T9s'), Hand('J2s'), Hand('J3s'), Hand('J4s'), Hand('J5s'), Hand('J6s'), Hand('J7s'), Hand('J8s'), Hand('J9s'), Hand('JTs'), Hand('Q2s'), Hand('Q3s'), Hand('Q4s'), Hand('Q5s'), Hand('Q6s'), Hand('Q7s'), Hand('Q8s'), Hand('Q9s'), Hand('QTs'), Hand('QJs'), Hand('K2s'), Hand('K3s'), Hand('K4s'), Hand('K5s'), Hand('K6s'), Hand('K7s'), Hand('K8s'), Hand('K9s'), Hand('KTs'), Hand('KJs'), Hand('KQs'), Hand('A2s'), Hand('A3s'), Hand('A4s'), Hand('A5s'), Hand('A6s'), Hand('A7s'), Hand('A8s'), Hand('A9s'), Hand('ATs'), Hand('AJs'), Hand('AQs'), Hand('AKs'))¶Tuple of suited hands in ascending order.
Combo¶
poker.hand.
Combo
[source]¶Hand combination.
See Combo
first
¶Type: | poker.card.Card |
---|
second
¶Type: | poker.card.Card |
---|
shape
¶Type: | Shape |
---|
- classmethod
from_cards
(first, second)[source]¶
is_broadway
¶
is_connector
¶
is_offsuit
¶
is_one_gapper
¶
is_pair
¶
is_suited
¶
is_suited_connector
¶
is_two_gapper
¶
rank_difference
¶The difference between the first and second rank of the Combo.

to_hand
()[source]¶Convert combo to Hand
object, losing suit information.
Range¶
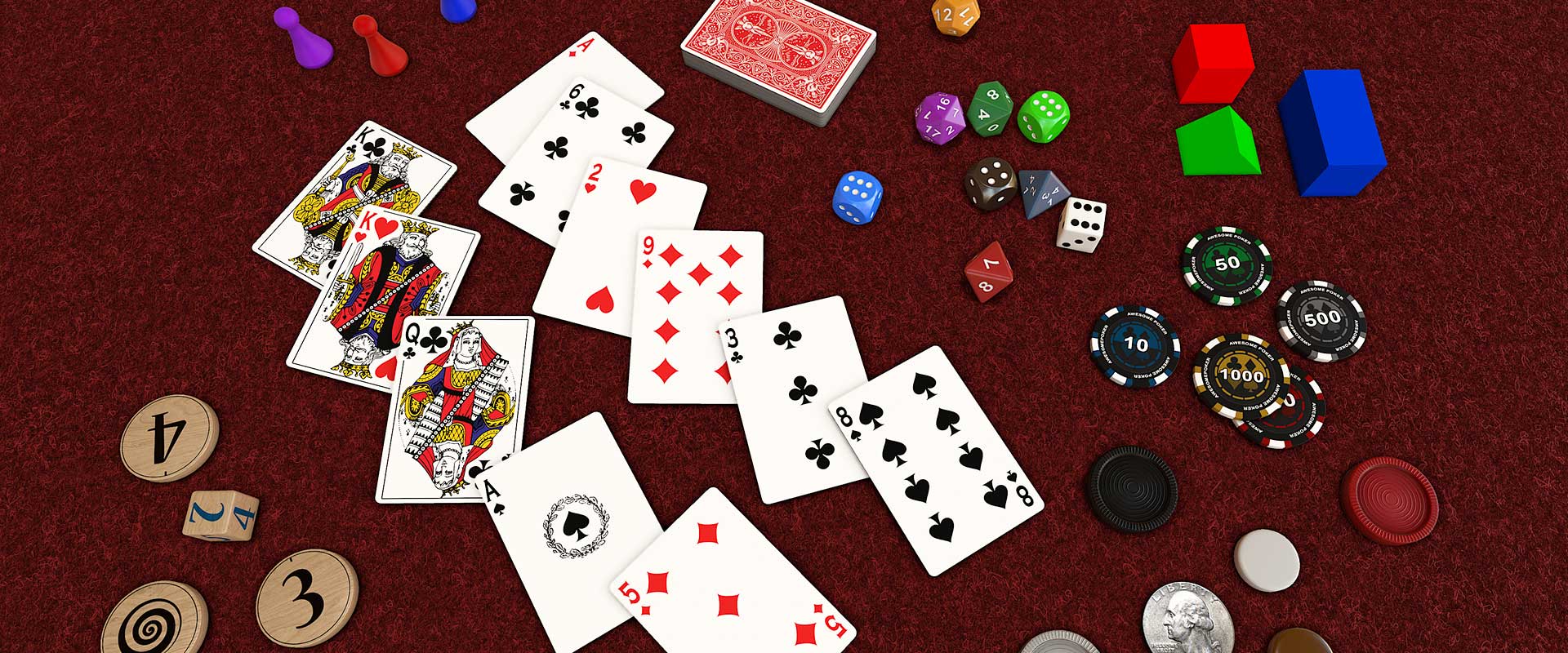
poker.hand.
Range
(range=')[source]¶Parses a str range into tuple of Combos (or Hands).
Parameters: | range (str) – Readable range in unicode |
---|
Poker Api Javascript App
Note
All of the properties below are cached_property, so make sure you invalidate the cache if you manipulate them!
hands
¶Tuple of hands contained in this range. If only one combo of the same hand is present,it will be shown here. e.g. Range('2s2c').hands(Hand('22'),)
Type: | tuple of poker.hand.Hand s |
---|
Poker Api Javascript Server
combos
¶Type: | tuple of poker.hand.Combo s |
---|
percent
¶What percent of combos does this range have compared to all the possible combos.
Poker Api Javascript Games
There are 1326 total combos in Hold’em: 52 * 51 / 2 (because order doesn’t matter)Precision: 2 decimal point
Type: | float (1-100) |
---|
rep_pieces
¶List of str pieces how the Range is represented.
Type: | list of str |
---|
to_html
()[source]¶Returns a 13x13 HTML table representing the range.
The table’s CSS class is range
, pair cells (td element) are pair
, offsuit hands areoffsuit
and suited hand cells has suited
css class.The HTML contains no extra whitespace at all.Calculating it should not take more than 30ms (which takes calculating a 100% range).
Return type: | str |
---|
to_ascii
(border=False)[source]¶Returns a nicely formatted ASCII table with optional borders.
Return type: | str |
---|
Poker Api Javascript Games
from_file
(filename)[source]¶Creates an instance from a given file, containing a range.It can handle the PokerCruncher (.rng extension) format.
from_objects
(iterable)[source]¶Poker Api Javascript Download
Make an instance from an iterable of Combos, Hands or both.
Poker Api Javascript App
slots
= ('_hands', '_combos')¶